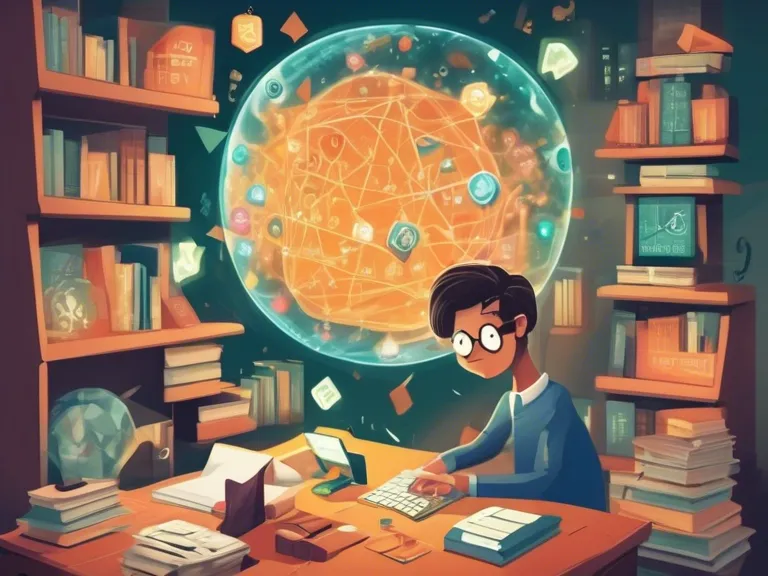
Introduction
Programming languages are like vast oceans teeming with hidden gems waiting to be discovered by curious developers. These hidden gems, also known as programming mysteries, are unique features, functions, or tricks that are not widely known but can significantly enhance a developer's productivity and code quality. In this article, we will delve into the world of programming mysteries and explore some of the hidden gems found in various programming languages.
Python: List Comprehensions
Python, known for its simplicity and readability, offers a powerful feature called list comprehensions. List comprehensions allow developers to create lists in a concise and elegant manner. Instead of writing traditional loops to iterate over elements, developers can use a single line of code to generate lists based on existing ones. This not only improves code readability but also reduces the number of lines of code required to achieve the same result.
# Example of list comprehension in Python
squared_numbers = [x**2 for x in range(1, 6)]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
JavaScript: Destructuring Assignment
JavaScript, the language of the web, offers a powerful feature called destructuring assignment. This feature allows developers to extract values from arrays or objects and assign them to variables in a simple and concise manner. Destructuring assignment can greatly simplify code that deals with complex data structures, making it easier to work with arrays and objects in JavaScript.
// Example of destructuring assignment in JavaScript
const person = { name: 'John Doe', age: 30 };
const { name, age } = person;
console.log(name, age); // Output: John Doe 30
SQL: Common Table Expressions (CTE)
SQL, the language for managing relational databases, offers a powerful feature called Common Table Expressions (CTE). CTE allows developers to define temporary result sets that can be referenced within a query. This feature is particularly useful for breaking down complex queries into more manageable parts, improving query readability and maintainability.
-- Example of Common Table Expression in SQL
WITH sales_data AS (
SELECT product_id, SUM(quantity) AS total_sales
FROM sales
GROUP BY product_id
)
SELECT product_id, total_sales
FROM sales_data;
Conclusion
Exploring programming mysteries and discovering hidden gems in various programming languages can not only enhance a developer's skills but also unlock new possibilities in code development. By leveraging these unique features and functions, developers can write more efficient, readable, and maintainable code. So, next time you delve into a new programming language, remember to keep an eye out for these hidden gems waiting to be discovered. Happy coding!
agoraguajara.com
amidstbooks.com
amoremagicallife.com
appspcdb.com
belatone.com
bistrotletranger.com
blackmountainfirearms.com
casollo.com
castlewoodlion.com
centromeninos.com
cubedentertainment.com
freestone-media.com
gargleblastrecords.com
geesent.com
glimmernews.com
iifingersbar.com
jean-samuel.com
kamakura-qs.com
liminal-order.com
mdgolden.com
monkeychop69.com
telegraphik.com
thmaniah8.com
ulf-mask.com
ulfbiotech.com
best-shoopping.com
blutreestudios.com
chateaumaplewood.com
dulichnguyenhung.com
fordlawfirmpllc.com
gandtblog.com
iwishihadapenguinfriend.com
kbresre.com
kimihime.com
manggih.com
marcelogoes.com
mustlovedanesrescue.com
nangluong-mattroi.com
nicolemidwest.com
paulinevknguyen.com
seleneboutiquesorrento.com
shanghai-culture.com
singturmg.com
thefantasyguide.com
theholygeek.com
thepressshopnyc.com
transportreloaded.com
whereeverigo.com
askchrisknight.com
askchristopherknight.com
berkshirefoodjournal.com
cchirosw.com
coastalcottagesnl.com
destinationsite.com
finulldraft.com
gardenstatepaintingnj.com
impacthound.com
inneddi.com
itazurakoneko.com
judyforaz.com
localcarsnow.com
lovedayscinema.com
matiqistiqomahsambas.com
meetborofive.com
morskicovik.com
naturesrewardphotography.com
regenerativeresorts.com
restaurant-nashvilletn.com
scoopsicecreamtruck.com
simmonsmotorworks.com
trinityeco-tours.com
uncoverstudios.com
wong-multimedia.com
123exp-government.com
abozymes.com
atsheatingandair.com
bemjavim.com
caseintrend.com
cdnxbvn.com
charangolibre.com
denis-latin.com
frugal-science.com
fukuoka-kouyaren.com
hanabisou.com
herculessportcenter.com
highvoltageloja.com
hlwstoryboard.com
juderabig.com
leauxandbehold.com
melurix-next.com
moggiechicken.com
mytrendysins.com
nativeskinonline.com
ninethreestudio.com
pcmwereld.com
pichinkufibers.com
primerautobody.com
salmanhatta.com
shalevbrosh.com
sosorryrecords.com
sportsone-egy.com
wofome.com
ycadeau.com
abalawpc.com
airportpawnmiami.com
alchemycollaborative.com
blackfigdesigns.com
cubemedica.com
deliciousyarns.com
denledamtrancaocap.com
denledpanelcaocap.com
futebolrico.com
honsyuji.com
jayasupranaschool.com
jstact.com
kikichaos.com
knuckleupmartialarts.com
lavi-furniture.com
maisonsecomatiq.com
neighbors-construction.com
nspointers.com
opticiwear.com
richouli.com
soddydaisyhealthcare.com
soultalkmagazine.com
studywithpmouy.com
swordfightsandstarrynights.com
tamatur-bali.com
thepainreliefsecret.com
townhouselinens.com
vchainstore.com
vormaro.com
yukonlodgeforsale.com
airkoolny.com
an-geeke.com
bajka3.com
bertinoargenti.com
birolkitabevi.com
burikoland.com
emeraldseamless.com
floridanativecharters.com
iptvsportt.com
jupiterboutique.com
kateronline.com
kustomkoachrv.com
lademeuredufondateur.com
lucianamurta.com
luckydelaware.com
luckyindiana.com
lujannavas.com
mike4rep.com
milioshairsalon.com
movingrestaurantequipment.com
openseedvault.com
retrogradetours.com
revistaresiduos.com
roughandreadygrange.com
therusalka.com
toxnfill3.com
valleymaintenancelandscape.com
violetorlandi.com
wanpaku-golf.com
zinkdental.com
suryacctv.com
tuvannugioi.com
mandalynmusic.com
wireless-reviews.com
tussingblockwatch.com
greenteaphotography.com
jennierooney.com
vietamreview.net
kyavar.com
market-truth.com
alexandregaurier.com
omegagadget.com
banditosla.com
nursememama.com
oyunbilim.com
acme-nuclear.com
agilityimap.com
akikcombong.com
anniesmysteries.com
bflofoodie.com
brandzonestudios.com
cacemar.com
daperezlaw.com
denvyautomation.com
eugeneband.com
factory-eshop.com
florentdumas.com
hishaywireless.com
in2-signs.com
kuwekeza-holdings.com
mitaniya-ltd.com
mixfoure.com
mobilitypluspro2.com
moipravila.com
montreal-business-kit.com
mortiseandmiter.com
nextdigitaldental.com
nurdalilahputri.com
oem-phoneaccessories.com
palmbeachestepona.com
precavida.com
roscoeandetta.com
scriptsnmacros.com
sringserver.com
thecustomfairy.com
withlovefromangela.com
applebyandwood.com
auzigog.com
eac-w.com
homesbyelevation.com
nihilismforoptimists.com
slavonkandhortus.com
thekoreanpolitics.com
turningpointpt.com
val-up.com
wakansen.com
3dideation.com
achilles-fire.com
banatelhalal.com
biyografirehberi.com
bohams.com
comisiondeestudios.com
cooride-net.com
danayumul.com
ecadecom.com
edwardscornerfarmersmarket.com
ekspresif.com
ellajmae.com
ginroecooks.com
gracefueled.com
hightidekitchen.com
jeroenswolfs.com
marthalott.com
mollybroekman.com
mpthoidai.com
plumfashionpr.com
racktents.com
solzapower.com
southcoastbehavioralhealth.com
the101bali.com
thearguide.com
theartistfia.com
thefitnesswire.com
thelivelihoodproject.com
thelynndentonagency.com
wilkespoolsnspas.com
wjwatson.com
drinkganbei.com
mendenhallnews.com
nathaliemoliavko-visotzky.com
nationalinfertilityday.com
wide-aware.com
ashleymodernfurniture.com
babylonbusinessfinance.com
charliedewhirst.com
christianandmilitaryhats.com
hypnosisoneonone.com
icelandcomedyfilmfestival.com
kayelam.com
mlroadhouse.com
mumpreneursonline.com
posciesa.com
pursweets-and.com
rgparchive.com
therenegadehealthshow.com
travelingbitz.com
yutakaokada.com
22fps.com
aarondgraham.com
essentialaustin.com
femdotdot.com
harborcheese.com
innovar-env.com
mercicongo.com
oabphoto.com
pmptestprep.com
rmreflectivevest-jp.com
tempistico.com
filmintelligence.org
artisticbrit.com
avataracademyagency.com
blackteaworld.com
healthprosinrecovery.com
iancswanson.com
multiversecorpscomics.com
warrenindiana.com
growthremote.com
horizonbarcelona.com
iosdevcampcolorado.com
knoticalpr.com
kotaden.com
la-scuderia.com
nidoderatones.com
noexcuses5k.com
nolongerhome.com
oxfordcounselingcenter.com
phytacol.com
pizzaropizza.com
spotlightbd.com
tenbags.com
thetravellingwilbennetts.com
archwayintl.com
jyorganictea.com
newdadsplaybook.com
noahlemas.com
qatohost.com
redredphoto.com
rooms4nhs.com
seadragonenergy.com
spagzblox.com
toboer.com
aumantvmuseum.com
beyondausten.com
citylabstudio.com
diskonio.com
drinkcf.com
eft-dongle.com
emilymeganphotography.com
evolveathleticclub.com
godleystationvet.com
hirochanweb.com
homeonefurniture.com
ifiwasastylist.com
lacantinepopup.com
liriklagubatak.com
lo-ko.com
mensagenseatividades.com
myway-zeus.com
nevadadec.com
nokarikhabar.com
nuuuki.com
quenchpad.com
sckyrock.com
tindunghanoi.com
tradeshows-biz.com
wikimuzik.com